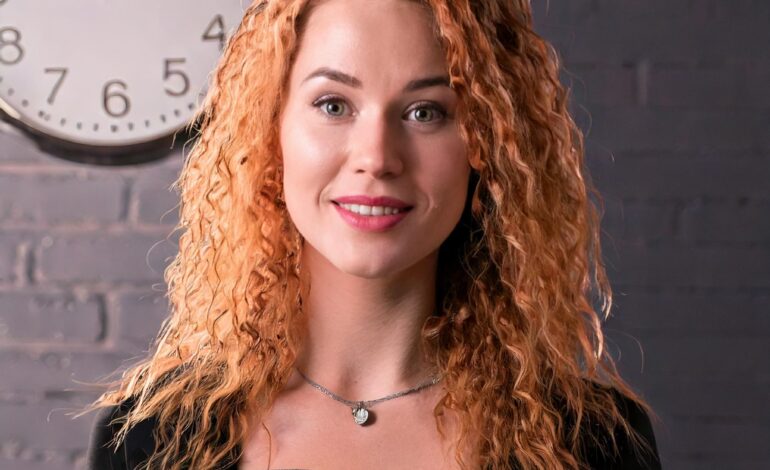
How to Become a Successful Angular Developer: A Step-by-Step Guide
Introduction
Angular Developer now days a lot of Developers have started using Angular, it has established itself as one of the go-to frameworks for creating single-page applications. Seeing its development or its importance is crucial for people planning to move in web development forward. This tutorial defines a structured approach towards learning Angular.
The person has to go through a few steps:
- Since Angular is based on TypeScript, it is important to first grasp the fundamentals of TypeScript.
- Getting acquainted with the Angular framework, its various architectural components, core concepts and modules.
- Developing ASD projects and working on other open source projects.
- As Angular releases a lot of updates it is important to be go through those and community work as well.
By using and going through the tutorial along these steps, Developers will be prepared for the fierce competition in the market throughout.
Angular in a Nutshell
Angular consists of a custom architecture that every Developer needs to understand. This architecture has interdependent components and constructs which are as follows:
- Modules: Each Angular application revolves around a module or modules; the decorator provides a better design structure for grouping related code.
- Components: Components are created through the use of logic, HTML and CSS as building blocks and are all related to a template which is assigned to each component.
- Services and Dependency Injection: With services, common provided functionalities across a set of components reach a wider audience with the use of dependable dependency injection that Angular has.
- Routing: In case of single page applications built with Angular, the Angular Router is what allows movement from one view or component to another.
- Directives and Pipes: Directives add more to HTML attributes while pipes are helpful in changing the form of the data so that it can be displayed in a certain way.
TypeScript for Angular, the New Beginning
A beginner who masters TypeScript targeting Angular should always start from the basics and keep advancing. This is how the roadmap looks like:
Basic Syntax and Typing:
- Grasp the methods of declaring variables, understanding the data types and type inference.
Interfaces and Classes:
- Investigate the means of writing interfaces and classes focusing on access modifiers and inheritance.
Generics:
- Develop the art of creating generic components to be reused.
Asynchronous Programming:
- Examine into the wide topic such as promises and a sync await and their relation to angular services.
Decorators:
- Be acquainted with angular decorators and krater’s , properties and the full use of Metadata.
Once these areas are conquered, the other crucial type of skill related to angular development using typescript will be developed.
Angular Developer Component Based Development
The angular framework has Component based development as a fundamental. It makes it possible for the developers to separate the graphical user interface into independent and reusable components. A component here is made up of HTML, CSS, and TypeScript codes.
Key Steps:
- Create Components: Components can be created by the angular CLI.
- Template Binding: Use interpolation and directives to bind properties of the component to the specific templates.
- Component Interaction: Structure the communication of the parent component and the child component using the Input and Output decorators.
- Styling: Style the components by applying encapsulated styles through one of Agular’s strategies for encapsulation.
- Lifecycle Hooks: Use the life cycle hooks like ‘nonfinite’ and ‘nonchanged’ provided by Angular.
Understanding these concepts enables building applications that can easily be scaled up and managed.
Reactive Programming with Rexes
Also known Reactive Extensions for JavaScript, Rexes is useful when dealing with asynchronous streams of data. It is vital for an Angular developer to know how to use the Rexes to manage the flow of data.
- Observables: They form the basis of the protocol that allows the communication between publishers and subscribers.
- Operators: Use operators to perform mapping, filtering out, merging and other methods to observables.
- Subjects: This is an observable that can also set itself as an observer hence dual functionality.
- Schedulers: Use the schedulers such as sync Scheduler or queue Scheduler to control the concurrency.
Angular Developer State Management and Ngoro
Ngoro is a state management library, the primary aim of which is to manage state in Angular Applications using reactive paradigm.
Key Concepts:
- Store: It’s where everything saves concerning the state of the application.
- Actions: These are procedures that bring about a desired change in state once completed.
- Reducers: These are pure functions that take the state from the beginning and return it at the end after any event that changes the state.
- Selectors: These are a way of getting a piece of the state.
- Effects: This executes an operation which realizes an asynchronous task.
Best Practices:
- Single Source of Truth: All state information needs to be stored in the store.
- Immutable State: It is a rule to not mutate state directly by any means.
- Action Creators: Create actions using various utility functions.
- Modular Reducers: Decomposition of reducers should be done in order to improve readability.
- Testing: For every action, reducer and effects, write tests.
It is important that such practices are followed in order to achieve a sustainable and a growing state management pattern in Angular applications.
Effective Utilization of Angular CLI
There is no Angular developer that would not want to make use of the Command Line Interface, Angular CLI helps to;
- Create a new Angular projects.
- This command creates the necessary folder architecture and adds a basic setup for the project.
Component Generation:
- Create further components without hassle.
- Also helps creating services, modules and guards through additional ng generate commands.
Development Server:
- Use to establish a development server.
- Supports hot reload for easier testing for any bugs.
Tests and Builds:
- Type out unit tests.
- Set the project up for deployment through.
Angular CLI basics
Creating a new application my app creating my component generate serving the application serve running the unit tests run the build.
Angular Developer Routing and Navigation
For single page applications (SPA) built with Angular, routing and navigation are important aspects to create an intuitive user interface. So it is important to learn the Angular Router.
- How to set up routing with the Router Module: This involves configuring routes by specifying a path and the associated component for that path.
- Link view navigation with views by using the router Link directive.
- Control route entry and exit parameters by applying Can Activate and Can Deactivate guards.
- Load feature modules only when needed with the lazy loading feature.
- Use query parameters to input and fetch information.
- Use wildcard routes to manage non-existing defined routes.
Making components and modules usable across applications
Angular projects have complex architectures, and as such a to understand how to write application agnostic components to keep a clean codebase. All components need to comply with the Single Responsibility Principle (SRP) and only focus on a single action or task.
Important Point:
- Navigate to the directory component to manage app, and run cli command to create components.
- There is bid to functional encapsulation alongside a module system.
Binding Methods:
- Apply Input and Output decorators that allow parent and child interaction.
- Two way binding could work as well with dynamic forms.
Services and Dependency Injection:
- Specify services across modules and pass them to components.
- Make use of Singleton services in case of need of shared data and logic.
Module Management:
- Group related components into feature modules.
- Use imports and exports of Modules to allow architecture to scale.
Note: With the help of reusable components, productivity is enhanced by eliminating the recurrence in large applications.
Performance Optimization Techniques
The best angular developers use a few performance optimization techniques in their Angular applications to make it work more efficiently.
- Lazy Loading: Place the load for the module dependencies at lazy sub module instead of load all.
- Ahead-Of-Time (AOT) Compilation: Compiling angular templates in advance to save up rendering time required.
- Track By with Angular Developer: Use tracking functions for filtering large lists when constructing.
- Tree-Shaking: When constructing the application, strip out code that is no longer in use.
- Service Workers: Allow for caching of content so that subsequent loads are more efficient and for times when a connection to the internet is not available.
- Reduce File Sizes: Optimize images and minimize files that contain CSS and JavaScript.
- Optimize DOM Access: Restrict the use of procedural interface manipulation of the document object model for efficiency.
Angular Developer Unit Testing and Debugging
Debugging and unit testing which are key development practices as for an Angular developer especially must be great at, if that developer wants the application to be robust and maintainable.
Familiarize oneself with Angular Testing Frameworks:
- Jasmine in the unit tests.
- E2E testing uses Protractor.
Install Testing Components:
- Add the test runner Karma where required.
- Provide Angular Testbed for the test.
Refer to Logical Unit Tests:
- Pay attention to units as an individual unit.
- Test using structuring blocks ‘describe’, ‘it’ and so forth.
Techniques for debugging:
- Angular CLI has flashing commands that aid in debugging.
- Direct manipulation in the effect as done within the application in Angular; addition tools are the gabbling tool.
Attempt to Test Integration:
- Link the projects with a CI/CD pipeline with the endpoints where tests are to be automated.
- Integrate Jenkins, GitHub or similar tools.
Angular Developer Implementation policies and coding standards
It is vital to adhere to coding policies and best practices to ensure that the developed application is scalable and maintainable.
- Angular Style Guide: Ensure compliance with the official guide to avoid inconsistencies.
- Understanding Component Based Application: Split the application into functional pieces wherever possible.
- Do not mix templates with business logic: Templates do not have features and should not contain template logic, hence, controllers help with it.
- Test Coverage: Will use Jasmine unit testing library and E2E testing with Protractor.
Evolving with the Angular Ecosystem Angular Developer
For a developer, remaining relevant with the Angular ecosystem is very important and critical for success. Here are crucial ways to keep you on the know:
- Read Blogs on Angular: Make it a habit to visit sites such as Angular Blog, In Depth.dev, or even Scotch.io on a regular basis.
- Participate in Angular Events: Active participation in events like ng-cone, Angular Connect, or attending sessions of local Angular groups.
- Social Media follow Angular celebrities: Follow the likes of Minco Gauche, Brad Green, and Kara Erickson on Twitter to name a few.
- Join Development Angular Developer: Other than Reddit/r/Angular2, participate in the discussion on Stack Overflow.
Conclusion: What’s Next in Angular Developer?
Now, for someone who wishes to learn Angular must only focus at coding and learning new code/patterns when necessary.
Key Actions:
- Join Communities: Be an active member of forums and social media pages.
- Open Source Contribution: Assist with open-source Angular projects.
- Get Coding: Do coding exercises and work on small coding projects.
- Certifications: Do not shy away from getting Angular certifications, it helps when looking for a job.
Additional Resources:
- Courses: There are several courses available on different e-Learning platforms such as Coursera, Udemy and Pluralsight.
- Blogs: Subscribe to popular blogs dedicated to Angular to get more information and news.