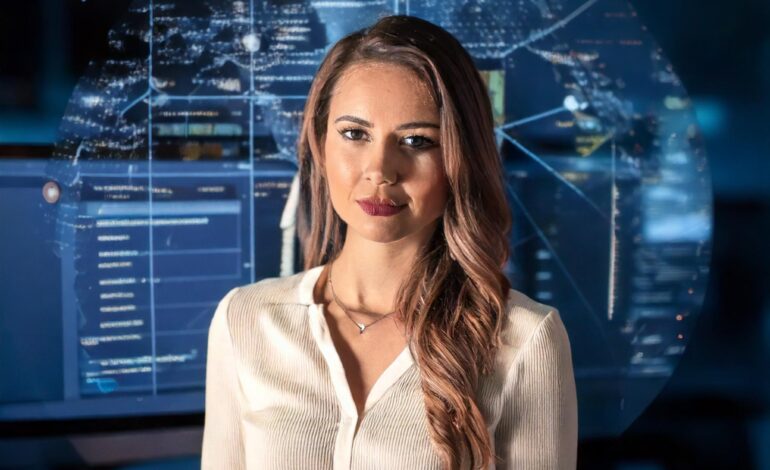
Why Hiring a React Developer is Essential for Your Next Project
Introduction
Facebook from 2000 onwards, React is a powerful open source JS tool, which is most popularly used when creating single-page applications. React will allow a developer to make single-use UI modules which are quite easy to use hence enhances efficiency of the whole process and increases maintainability further reducing the need of constantly rewriting the same code.
The most important features are:
- Component Based Architecture: This architecture organizes the UI into components, which makes it easier to create develop and test the application.
- Virtual DOM: This approach plays a crucial role in boosting rendering speeds by reducing how many DOM operations are necessary.
- Declarative Code: This eye-friendly feature aids in debugging and code comprehension because it enables developers to communicate the UI’s state rather than explaining how the code should function.
Any developer wonders, in what areas does React excel in?
- Performance: The time taken by such updates and render processes from React are efficient.
- Scalability: For an application having thousands of users, needs to be large.
- Community Support: The broader the community the more the third-party libraries.
ES6 and the React Developer understanding of JavaScript
In order for a developer to be a proficient React developer, one needs an extensive understanding of JavaScript and also other recent updates in ES6+ features, as this will enable one to write effective code.
Some key considerations include the following:
- Arrow Functions: Instead of specifying it every time it says this: function all this complexity comes down to the symbol.
- Classes: High-level syntax to do object-oriented design using methods along with inheritance.
- Modules: Helps segregate the code into smaller sections, so you can import and export the relevant codes making it easier to code.
- Destructuring: Breaking down any array or object into individual set of variables or properties.
Include everything as it all makes sense:
- Promises and Async Await: Improves handling asynchronous code making it easy to read and comprehend along with error handling.
- Template Literals: This is how easily you can embed expressions when string interpolation takes place.
Flawless handling of these features guarantees code quality and processes optimization.
Introduction to Component Based Architecture and JSX
JavaScript can be enhanced using JSX which allows for the integration of HTML code inside of the React framework. It makes the creation of React elements easier and increases the readability of the codes. Component Based Architecture allows for the creation of reusable encapsulated building blocks improving modularity and making future maintenance easier.
Discussed here first
Benefits of JSX:
- Enhances how code is read
- Direct integration of HTML in Javascript
Component-Based Architecture:
- Component patterns are easier to use
- Diagnostics and validation are more effective
“A JSX element is simply an object representation of HTML elements and custom React components…” -React Official Docss.
Implementing State Management in React Developer
When dealing with ever changing data, managing state in React is of utmost importance. React has a number of features for state management:
- useState Hook: it allows functional components to have state variables.
- Context API: enables semi-global variables to be used easily across components.
- useReducer Hook: It is used to implement complex state management and behaves like Redux.
- Redux: It is generally a state management library for greater applications with the advantage of being predictable.
One of the essential concepts needed to make the user interface seamless is managing the state. Apart from facilitating debugging, this aspect also allows for the codebase to be easily maintained. Involving the use of hooks and state libraries makes this state management both easy and in turn boosts the application’s efficiency.
Basics of React Hooks React Developer
Everybody knows that a good React developer has to know how the React Hooks work. Hooks were released along with a version update to 16.8 and were aimed at allowing functional components to have state and lifecycle methods which before this could only be done in class components. Some of the essentials include:
- UseState: This allows for maintaining a local state inside function components.
- UseEffect: This manages operations like data fetching or subscriptions.
- UseContext: This makes it easier to pass the values around through components instead of manually placing them as props.
Also, learning the advanced hooks is important:
- UseMemo & Use Callback: They aim in increasing performance by caching the values and functions.
- UseReducer: This is used when managing complex use cases instead of using useState for state management.
Using React Router to Anchor Client Side Navigation
React Router was designed to integrate features that allow users to switch between the pages in a single-page application. Thanks to the dynamic routes that it offers to the users, it allows the use of multiple views without having to refresh the page.Some key features include:
- Declarative Routing: With the use of JSX, it’s much easier for developers to specify routing which in turn makes the code cleaner.
- Nested Routes: The integration of nested routes allows the developers to build complex UIs with multiple layers more easily.
- Dynamic Routing: Navigation can change depending on what’s happening in the app when a user is interacting with it.
- Code Splitting: It is an optimization that allows for code that corresponds to a particular route to be fetched only when that route is displayed.
- Browser History Integration: It establishes a pairing between the user interface and the navigation action within the web browser, keeping a history record.
To have a good experience while using the application, a React developer is expected to have knowledge of React Router.
CSS in JS and Styling Approaches React Developer
With React being the part of modern web development, styling in CSS-in-JS seems to be the go to option. For your understanding, here’s the reason why:
- Scoped Styles: Defined styles are applied to specified components without affecting other global CSS rules.
- Dynamic Styling: Style can be changed based on the status of the component and the conditions set.
- Maintainability: Since modular styles assign CSS for each component, it is much easier to maintain and scale.
- Performance: Critical rendering path is streamlined by only rendering the essential CSS and leaving out the non crucial parts.
- Integration: Goes along with JavaScript well as there are easy ways of incorporating props, states and themes.
“It Allows CSS styling with React using a component approach. This increases overall productivity and improve maintainability”
Building Forms and Handling User Input
React developers make it look seamless to work with creating complex forms and also managing the data that the forms collect.
- Componentization: For forming purposes, React developers can design form fields that can be reused.
- State Management: The form states are efficiently controlled through the use of the useState and useReducer Hook.
- Validation: Formik and Yup can validate with strong accuracy via integration into the forms.
- Controlled Components: Use controlled components to have better control on the form elements.
- Accessibility: Allows forms to be usable, which conforms to WCAG.
Utilizing React’s virtual DOM allows a fluid and responsive interaction with the forms and improves the experiences of the user.
This experience guarantees robust forms and easy forms as well.
Testing and Debugging in React React Developer
Testing and debugging in React are highly specialized jobs. Many tools developers of a React application use to create a strong application:
- Jest: Makes it possible to do unit testing of a single component to test whether it works properly without being combined with the others.
- Enzyme: Helps in carrying out testing of the output of components.
- React Testing Library: Promotes user centered testing.
- ESLint: Enables searching for an issue in the code that does not comply with the rules and standards of code writing.
- Redux DevTools: Aids in tracking down bugs regarding state changes in Redux.
By employing a skilled React developer, you know and can be sure that these tools were well used, providing a great and well-tested application. A skilled developer will also ensure that bugs will be reduced and the debugging processes will be more efficient.
Optimization Techniques and Performance Best Practices
There are also crucial factors that one should consider before hiring a React developer, such as optimization techniques and performance best practices.
Areas that can be optimized:
- Code Splitting: Implementing dynamic import so that components are loaded when necessary.
- Loading Sections: Such approach reduces cumbersome tasks like loading images or components viewable off the screen when the user is not scrolling.
- Memoization: The memoization of data is created by observing approaches that are adopted to minimize unnecessary renders.
- Virtualization: The use of libraries like intricacy of working with large lists.
- Optimization Tool: Improving bundles by utilizing tools such as Webpack.
Performance Packed Measures:
- The goal should be simple try to have the least number of stateful components in your system.
- Utilize for more controlled re-rendering rather than different approaches which are less efficient.
- Getting a production build ready and available for your deployment should be a priority.
- Always consider React DevTools for profiling or auditing a function.
- One of the best options available when optimizing is SSR as it directly has been shown to reduce load time.
Any APIs or Asynchronous Data a React Developer
API management is an area that React developers excel at as it’s a crucial aspect of any new application. As this is a place this tends to make or break applications, APIs enable interaction with servers, databases and upholds connections with other third party systems. Therefore their skills incorporate the following;
- Getting Information: Getting things in order using either fetch API or Axios for any HTTP request going forward.
- Responding Back: In this case items such as JSON parsing and states with stateful components or different hooks.
- Making Errors: This will include an implementation of try/catch blocks or an error boundary, along with appropriate messages for users.
- Asynchronous Requests: Getting the necessary data using async/await or promises should be the way to go.
- Data Handling: Here context API or Redux should be leveraged for better and consistent state across different components.
- Efficient Resource Usage: Using React.memo and lazy loading will improve performance across your application.
Version Control via Git and Team Work
Using Git for the project versioning ensures that all the changes done by team members to the project are recorded and they can be restored when need be. This enhances visibility and enables all team members to see what has been achieved so far.
- Branch Handling: Developers are able to use different branches in order to enable them work on feature additions and fixes independently.
- Code Review: Changes in code are made to be viewed and accepted via a pull request which improves coding or practices and collaboration.
- Dealing with Conflicts: Git assists significantly in the locating and fixing of merging work alteration conflicts.
- Change Tracking: Keeps records for all modifications that have been done thereby facilitating understanding and monitoring of changes, for example, the development of the code.
- Integration Testing: Is possible to join with the CI/CD pipelines for automated tests and deployments.
What is Continuous Integrations and Continuous Deployment?
Continuous Integrations and Continuous Deployments (CI/CD) is also generally considered the heart of software development today. With CI/CD in mind, the changes a React developer makes will reflect throughout the system efficiently and without any mistakes.
Such updating processes benefits:
- Easy to handle and use: Cuts down the need of manually running tests and deployments.
- Efforts in all tasks: Helps in a wide range of areas while increasing chances of errors during a build.
- Less progress and time management: Supports having many tests and deploying them faster, while waiting for the changes to show less time.
Some must-have abilities that a React Developer should have:
- Source Control Management: Solid skills of Git/GitHub working within the projects.
- Build Programs: Highly proficient with Webpack or Babel or any other fill in the blank programs.
- Testing Frameworks: Knowledge of Jest, Mocha for writing automated tests is a bonus.
- CI/CD Services: Has worked with Jenkins, Travis CI or GitHub Actions.
“Automation is good, as long as you know in advance the places where it could be applied.”
Following the Evolution of React Ecosystem and Community
As a React developer’s role is very important to keep pace with the progressive ecosystem and the community, the React domain consists of:
- Libraries and Tools: Extensive knowledge about crucial libraries like Redux, React Router and Styled Components.
- Best Practices: Familiarity with the best practices that are in use today such as hooks, context API and state management.
- Community Contributions: Participation in the community, forums, repositories on GitHub, and React conferences.
- Framework Updates: Self-training on the new versions and features of the React framework.
- Performance Optimization: Familiarity with techniques such as code splitting and memoization for purposes of performance optimization.React is already an established and effective ecosystem, to say the least, a good developer makes sure that your application is bang up to date with the best developments.
Conclusion and Further Learning Resources
Getting a React developer comes with a lot of benefits to the project as they guarantee a speedy development process as well as a better user experience. To always keep abreast and further enrich understanding of the subject of interest, in this particular case React and its ecosystem, these resources might be useful:
- Official React Documentation: The Official Online Resource for concepts and API documentations of React.
- React Training: Provides courses and workshops on React-Structured Learning.
- Medium and Dev.to: Two sources where the industry is full of articles from the specialists.
- GitHub Repositories: Find relevant open-source products for fieldwork.
- Udemy and Coursera: Spanning the various aspects of a react developers work the courses are online.
- YouTube: There are examples of channels where such tutorials can be found like Channel Academind or Traversy Media.